![]() |
128x64 |
![]() |
16x2 |
![]() |
320x240 QVGA |
![]() |
16x1 |
There are so much brand in market. The famous one brand among students in university is Hitachi 16x2 Alphanumeric LCD module (refer specification). It can show display 16 bit output with 2 row which is equal to 32 bit in real time. We cannot just connect LCD to micro-controller because every each pin have the reserved function based on data sheet. Below is example pin configuration for 16x2 LCD module:
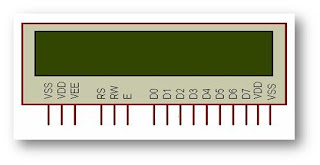
Pin
|
Symbol
|
Description
|
1
|
VSS
|
Ground
|
2
|
VDD
|
+5V Power Supply
|
3
|
VEE
|
Contrast Adjustment
|
4
|
RS
|
0: Command Register
1: Data register
|
5
|
RW
|
0: Write
1:Read
|
6
|
E
|
Enable
|
7-14
|
D0-D8
|
Data bus
|
15
|
LED+
|
Backlight Positive Power Supply
|
16
|
LED-
|
Backlight Ground
|
For circuit connection LCD to micro-controller has shown below:
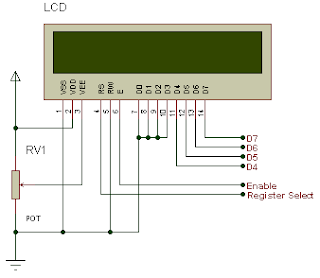
Figure : LCD module connection
As we can see, VSS is going for ground, VDD to +5V and VEE to contrast adjustment. Here we using variable resistor, so we can adjust how much brightness that we want. Other pin is refer to coding that we want to program. Some person say that pin D0-D7 should connect to port D on micro-controller, but exactly we can put it on port where we want. All this depends on our programming, whether we declare the variable at port C, port D or else.
Ok, lets move on to the programming method. Now is the part that need to understand carefully. Here the step before start the programming:
- Determine which port your want to use to connect with pin D0-D7 and RS, RW and E on LCD module.
- Connect circuit like picture above (except port that you have determine in step 1).
- Turn on power and see whether the LCD is function or not.
- If ok, go to step programming.
- Open MPLAB, and write programming.
LCD Progamming
To program the LCD with micro-controller is little bit tricky. You must need to read the datasheet and must know your compiler. Different compiler different style. Here i'm using C18 compiler since my previous project is using PIC18F family. Below show the simple programming for LCD with PIC:
/*Header******************************************************/
// LCD module connections
sbit LCD_RS at RB4_bit;
sbit LCD_EN at RB5_bit;
sbit LCD_D4 at RB0_bit;
sbit LCD_D5 at RB1_bit;
sbit LCD_D6 at RB2_bit;
sbit LCD_D7 at RB3_bit;
sbit LCD_RS_Direction at TRISB4_bit;
sbit LCD_EN_Direction at TRISB5_bit;
sbit LCD_D4_Direction at TRISB0_bit;
sbit LCD_D5_Direction at TRISB1_bit;
sbit LCD_D6_Direction at TRISB2_bit;
sbit LCD_D7_Direction at TRISB3_bit;
// End LCD module connections
unsigned char ch; //
unsigned int adc_rd; // Declare variables
char *text; //
long tlong; //
void main() {
INTCON = 0; // All interrupts disabled
ANSEL = 0x04; // Pin RA2 is configured as an analog input
TRISA = 0x04;
ANSELH = 0; // Rest of pins are configured as digital
Lcd_Init(); // LCD display initialization
Lcd_Cmd(_LCD_CURSOR_OFF); // LCD command (cursor off)
Lcd_Cmd(_LCD_CLEAR); // LCD command (clear LCD)
text = "mikroElektronika"; // Define the first message
Lcd_Out(1,1,text); // Write the first message in the first line
text = "LCD example"; // Define the second message
Lcd_Out(2,1,text); // Define the first message
ADCON1 = 0x82; // A/D voltage reference is VCC
TRISA = 0xFF; // All port A pins are configured as inputs
Delay_ms(2000);
text = "voltage:"; // Define the third message
while (1) {
adc_rd = ADC_Read(2); // A/D conversion. Pin RA2 is an input.
Lcd_Out(2,1,text); // Write result in the second line
tlong = (long)adc_rd * 5000; // Convert the result in millivolts
tlong = tlong / 1023; // 0..1023 -> 0-5000mV
ch = tlong / 1000; // Extract volts (thousands of millivolts)
// from result
Lcd_Chr(2,9,48+ch); // Write result in ASCII format
Lcd_Chr_CP('.');
ch = (tlong / 100) % 10; // Extract hundreds of millivolts
Lcd_Chr_CP(48+ch); // Write result in ASCII format
ch = (tlong / 10) % 10; // Extract tens of millivolts
Lcd_Chr_CP(48+ch); // Write result in ASCII format
ch = tlong % 10; // Extract digits for millivolts
Lcd_Chr_CP(48+ch); // Write result in ASCII format
Lcd_Chr_CP('V');
Delay_ms(1);
}
}
*source from mikroElektronika website.
Programming LCD screen is not simple as it look. We need to study a little bit about LCD background which can find in datasheet/ catalog and as a result practice make perfect.
No comments:
Post a Comment